In one of my previous posts, I tried to figure out what to do with subviews in SwiftUI. There's four ways of building subviews for SwiftUI:
- Building the subview embedded directly into the parent's body.
- This will usually happen for structural subviews (e.g. HStack or Vstack) and for simpler subviews.
- Building the subview as a new structure.
- Once the subview becomes more complicated, we need to break it apart, both to help with rendering and code structure. Building a new struct which conforms to View provides both of those benefits.
- Building the subview as a @ViewBuilder function or property of the parent view.
- This also provides both benefits of helping with rendering and code structure. The added benefit, however, is that we can mark this implementation as private more easily than creating a new struct.
- Building the subview as a non-@ViewBuilder property.
- As far as I can tell, there's no real use for this, unless the subview is completely static.
Now, I'm writing part 2 of this article because I realized that there is another big difference between points 2️⃣ and 3️⃣: previews.
Even though I still believe that building a subview as a @ViewBuilder function allows you to encapsulate views more, which is usually a good thing, providing a preview of the view alone is a bit more cumbersome. Surprisingly, SwiftUI doesn't actually mind us having more than one preview in a Swift file. The following shows the Canvas and how we have two different previews.
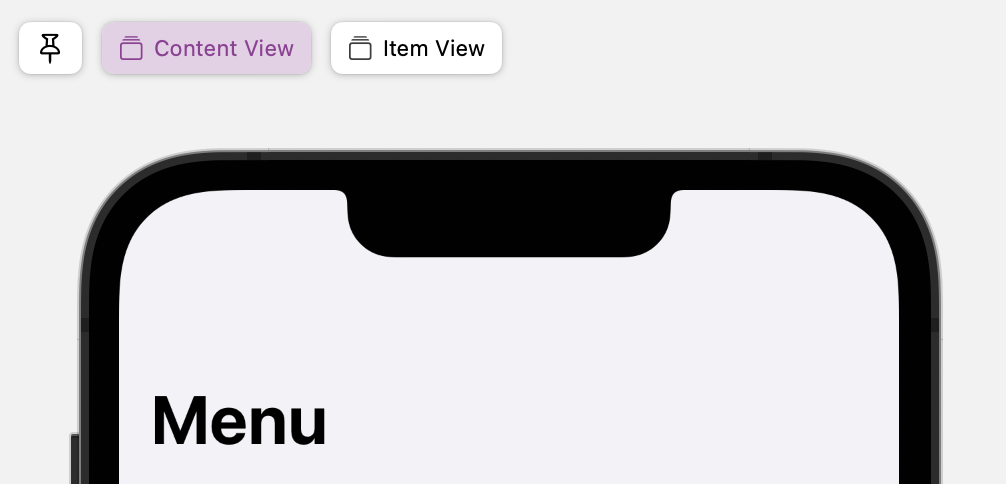
I guess in the end this then comes down to both preference and access control. The last remaining question is whether it'd be more performant to have a struct or a @ViewBuilder function. "Thinking in SwiftUI" by objc.io heavily hints that there would be no performance benefit since the render tree for the same view built in these two ways would not change.
We'll see.